Java如何操作Office办公软件
- 在工作中,大家应该有遇到,用代码去读取或导出Excel、WORD、PDF 这类的需求吧
- 如果有做过这方面的大佬,应该都了解过 Apache POI .
- Apache POI 是用Java编写的免费开源的跨平台的 Java API.
- Apache POI提供API给Java对Microsoft Office格式档案读和写的功能。
- 但是大部分人都是用其他的框架,因为POI比较耗内存,如果导出数据量大的话容易OOM
- 而大部分的其他框架都是基于 POI基础上再次开发的。
- 废话不多说,直接上代码
一、操作Excel
1、快速使用
二、操作Word
- 也可以用POI,但是更推荐使用:
Poi-tl
- Poi-tl 也是基于 POI 再次封装的。
- Github地址:https://github.com/Sayi/poi-tl
- pot-tl 主要用于 Word模板 导出,在模板中可定制多种类型的数据:包含有文本、图片、表格、列表、图表、等等。
- 有多个版本,使用不同POI 就引入不同的版本,对照表如下:
版本 |
POI版本 |
JDK版本 |
1.12.0 Documentation(当前最新版本) |
Apache POI5.2.2+ |
JDK1.8+ |
1.11.x Documentation |
Apache POI5.1.0+ |
JDK1.8+ |
1.10.x Documentation |
Apache POI4.1.2 |
JDK1.8+ |
1.10.3 Documentation |
Apache POI4.1.2 |
JDK1.8+ |
1.9.x Documentation |
Apache POI4.1.2 |
JDK1.8+ |
1.8.x Documentation |
Apache POI4.1.2 |
JDK1.8+ |
1.7.x Documentation |
Apache POI4.0.0+ |
JDK1.8+ |
1.6.x Documentation |
Apache POI4.0.0+ |
JDK1.8+ |
1.5.x Documentation |
Apache POI3.16+ |
JDK1.6+ |
1、快速开始
1 2 3 4 5
| XWPFTemplate template = XWPFTemplate.compile("template.docx").render( new HashMap<String, Object>(){{ put("title", "Hi, poi-tl Word模板引擎"); }}).writeAndClose(new FileOutputStream("output.docx"));
|
- 所有的标签都是以 结尾,标签可以出现在任何位置,包括页眉,页脚,表格内部,文本框等,表格布局可以设计出很多优秀专业的文档,推荐使用表格布局。
- 当我们需要遍历的时候就用区块对,
- 区块对由前后两个标签组成,开始标签以?标识,结束标签以
/
。
- 图片和图表的使用
- 图片和多系列图表的标签是一个文本:
{{var}}
,标签位置在:图表区格式—> 可选文字—>(新版本Microsoft Office标签位置在:编辑替换文字-替换文字)
。
- 我目前是新版本,所以位置在
替换文字
。
- 如下图:
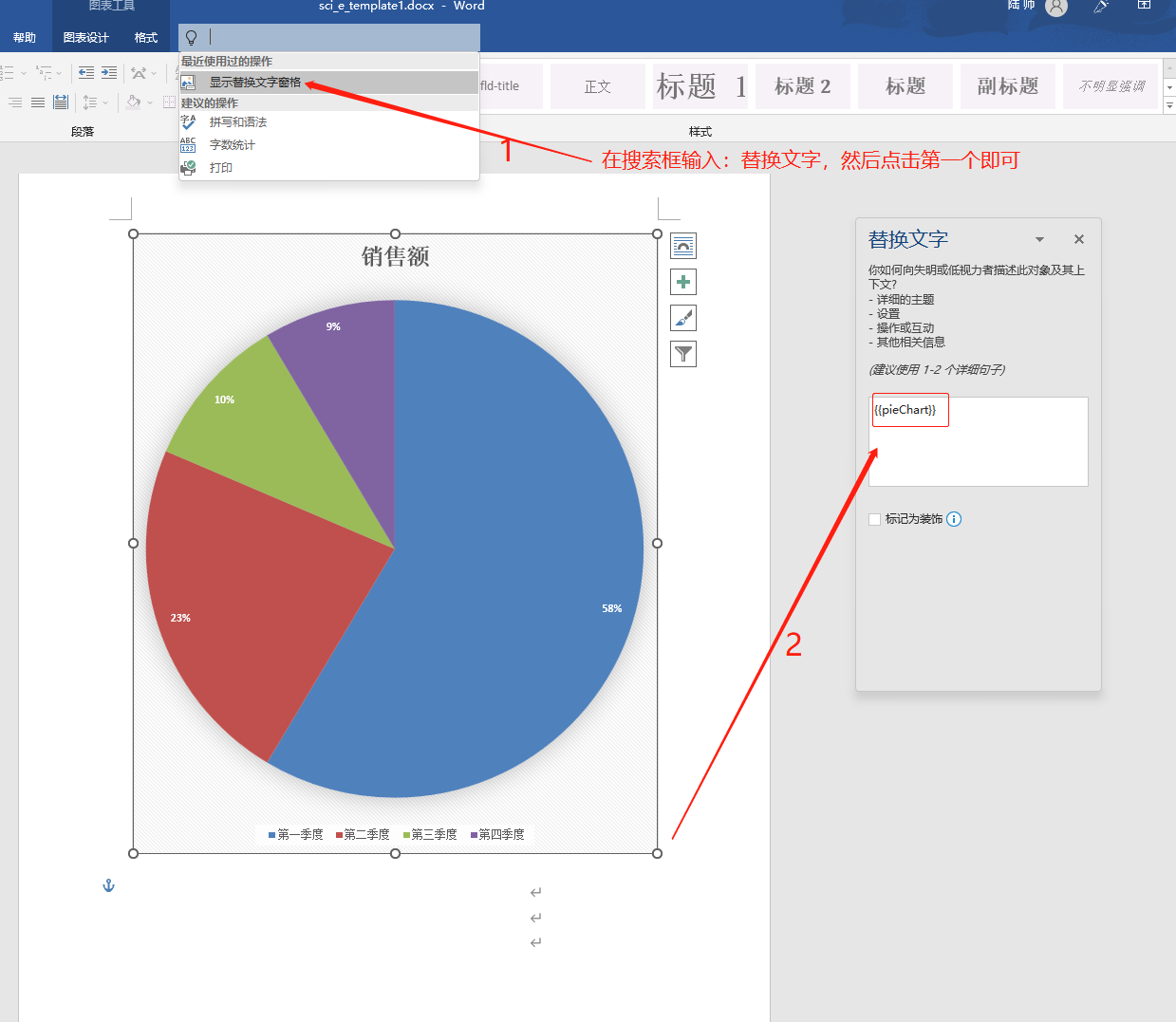
1 2 3 4 5 6 7 8
| ChartSingleSeriesRenderData pie = Charts.ofPie("编程语言分类", new String[] { "Java", "C++", "Go", "python"}) .series("countries4", new Integer[] { 888, 666,333,222 }).create(); XWPFTemplate.compile(templateFile).render(new HashMap<String, Object>(){{ put("title", "2022年7月"); put("pieChart", pie); }}).writeAndClose(new FileOutputStream("output.docx"));
|
三、操作PDF
- PDF一般导出功能也挺多的,如:HTML导出为PDF、word导出为PDF、excel导出为PDF等
- 其他我没用过我就不介绍了,我只用过excel导出为PDF。
1、Excel导出PDF
- 在网上找到一个使用:
aspose-cells-8.5.2.jar
包的。
- 这个没有可以用的pom地址,需要额外下载。
- 代码如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93
| import com.aspose.cells.License; import com.aspose.cells.PdfSaveOptions; import com.aspose.cells.Workbook; import java.io.FileOutputStream; import java.io.InputStream; public class Excel2Pdf { public static void main(String[] args) { String sourceFilePath="D:\\work_space\\文档\\产品需求文档\\文献收录情况检索报告参考模版(珠江医院图书馆).xlsx"; String desFilePath="D:\\work_space\\文档\\产品需求文档\\rest.pdf"; excel2pdf(sourceFilePath, desFilePath); }
public static boolean getLicense() { boolean result = false; try { InputStream is = Excel2Pdf.class.getClassLoader().getResourceAsStream("\\license.xml"); License aposeLic = new License(); aposeLic.setLicense(is); result = true; } catch (Exception e) { e.printStackTrace(); } return result; }
public static void excel2pdf(String sourceFilePath, String desFilePathd ){ if (!getLicense()) { return; } try { Workbook wb = new Workbook(sourceFilePath); FileOutputStream fileOS = new FileOutputStream(desFilePathd); PdfSaveOptions pdfSaveOptions = new PdfSaveOptions(); pdfSaveOptions.setOnePagePerSheet(true); int[] autoDrawSheets={3};
int[] showSheets={0}; printSheetPage(wb,showSheets); wb.save(fileOS, pdfSaveOptions); fileOS.flush(); fileOS.close(); System.out.println("完毕"); } catch (Exception e) { e.printStackTrace(); } }
public static void autoDraw(Workbook wb,int[] page){ if(null!=page&&page.length>0){ for (int i = 0; i < page.length; i++) { wb.getWorksheets().get(i).getHorizontalPageBreaks().clear(); wb.getWorksheets().get(i).getVerticalPageBreaks().clear(); } } }
public static void printSheetPage(Workbook wb,int[] page){ for (int i= 1; i < wb.getWorksheets().getCount(); i++) { wb.getWorksheets().get(i).setVisible(false); } if(null==page||page.length==0){ wb.getWorksheets().get(0).setVisible(true); }else{ for (int i = 0; i < page.length; i++) { wb.getWorksheets().get(i).setVisible(true); } } } }
|
- 其中的许可文件:
license.xml
内容如下:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| <?xml version="1.0" encoding="UTF-8"?> <License> <Data> <Products> <Product>Aspose.Total for Java</Product> <Product>Aspose.Excel for Java</Product> </Products> <EditionType>Enterprise</EditionType> <SubscriptionExpiry>20991231</SubscriptionExpiry> <LicenseExpiry>20991231</LicenseExpiry> <SerialNumber>8bfe198c-7f0c-4ef8-8ff0-acc3237bf0d7</SerialNumber> </Data> <Signature>sNLLKGMUdF0r8O1kKilWAGdgfs2BvJb/2Xp8p5iuDVfZXmhppo+d0Ran1P9TKdjV4ABwAgKXxJ3jcQTqE/2IRfqwnPf8itN8aFZlV3TJPYeD3yWE7IT55Gz6EijUpC7aKeoohTb4w2fpox58wWoF3SNp6sK6jDfiAUGEHYJ9pjU=</Signature> </License>
|
2、读取PDF
- 其实读取PDF的需求也算常见吧。
- 读取PDF一般用到:
pdfbox
或 e-iceblue
或 Aspose.PDF for Java
第一种:pdfbox
1 2 3 4 5
| <dependency> <groupId>org.apache.pdfbox</groupId> <artifactId>pdfbox</artifactId> <version>2.0.26</version> </dependency>
|
1 2 3 4 5 6 7
| public static void main(String[] args) throws IOException { PDDocument pdDocument = PDDocument.load(new File("Demo.pdf")); PDFTextStripper textStripper = new PDFTextStripper(); String text = textStripper.getText(pdDocument); System.out.println("内容="+text); pdDocument.close(); }
|
第二种:e-iceblue
1 2 3 4 5
| <dependency> <groupId>e-iceblue</groupId> <artifactId>spire.pdf</artifactId> <version>5.4.0</version> </dependency>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| import com.spire.pdf.PdfDocument; import com.spire.pdf.PdfPageBase; import java.io.*;
public class Extract_Text {
public static void main(String[] args) { PdfDocument doc = new PdfDocument(); doc.loadFromFile("test.pdf");
StringBuilder sb = new StringBuilder(); PdfPageBase page; for(int i= 0;i<doc.getPages().getCount();i++){ page = doc.getPages().get(i); sb.append(page.extractText(true)); } FileWriter writer; try { writer = new FileWriter("ExtractText.txt"); writer.write(sb.toString()); writer.flush(); } catch (IOException e) { e.printStackTrace(); }
doc.close(); } }
|
第三种:Aspose.PDF for Java
您的打赏,是我创作的动力!不给钱?那我只能靠想象力充饥了。
微信支付
支付宝